实践过程
效果
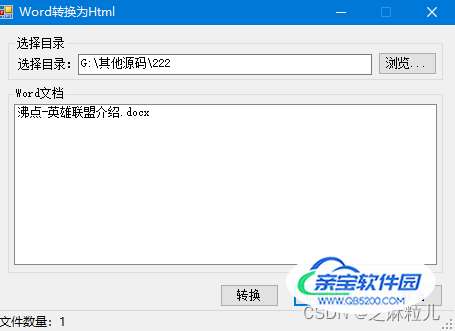
代码
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
public static void BatchConvert(string docDir)//如果批量转换
{
//创建数组保存文件夹下的文件名
string[] docFiles = Directory.GetFiles(docDir, "*.doc");
for (int i = 0; i < docFiles.Length; i++)
{
WordToHtmlFile(docFiles[i]);
}
MessageBox.Show("转换完毕!", "提示信息", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
//将word转换为html
public static void WordToHtmlFile(string WordFilePath)
{
try
{
Microsoft.Office.Interop.Word.Application wApp = new Microsoft.Office.Interop.Word.Application();
//指定原文件和目标文件
object docPath = WordFilePath;
string htmlPath;
if (WordFilePath.Contains(".docx"))
{
htmlPath = WordFilePath.Substring(0, WordFilePath.Length - 4) + "html";
}
else
{
htmlPath = WordFilePath.Substring(0, WordFilePath.Length - 3) + "html";
}
object Target = htmlPath;
//缺省参数
object Unknown = Type.Missing;
//只读方式打开
object readOnly = true;
//打开doc文件
Microsoft.Office.Interop.Word.Document document = wApp.Documents.Open(ref docPath, ref Unknown,
ref readOnly, ref Unknown, ref Unknown,
ref Unknown, ref Unknown, ref Unknown,
ref Unknown, ref Unknown, ref Unknown,
ref Unknown);
// 指定格式
object format = Microsoft.Office.Interop.Word.WdSaveFormat.wdFormatHTML;
// 转换格式
document.SaveAs(ref Target, ref format,
ref Unknown, ref Unknown, ref Unknown,
ref Unknown, ref Unknown, ref Unknown,
ref Unknown, ref Unknown, ref Unknown);
// 关闭文档和Word程序
document.Close(ref Unknown, ref Unknown, ref Unknown);
wApp.Quit(ref Unknown, ref Unknown, ref Unknown);
}
catch (Exception e)
{
MessageBox.Show(e.Message);
}
}
private void button1_Click(object sender, EventArgs e)
{
if (folderBrowserDialog1.ShowDialog() == DialogResult.OK)
{
tsslInfo.Text = "";
txtWordPath.Text = folderBrowserDialog1.SelectedPath;
string[] docFiles = Directory.GetFiles(txtWordPath.Text.Trim(),"*.doc");
for (int i = 0; i < docFiles.Length; i++)
{
string docPath=docFiles[i].ToString();
string docName = docPath.Substring(docPath.LastIndexOf("\\") + 1, docPath.Length - docPath.LastIndexOf("\\") -1);
listView1.Items.Add(docName);
}
tsslInfo.Text = "文件数量:"+listView1.Items.Count.ToString();
}
}
private void button2_Click(object sender, EventArgs e)//单个转换
{
if (listView1.Items.Count > 0)
{
if (listView1.SelectedItems.Count > 0)
{
if (MessageBox.Show("确定将此文档转换为HTML文件吗?", "提示信息", MessageBoxButtons.OKCancel, MessageBoxIcon.Information) == DialogResult.OK)
{
string docPath = txtWordPath.Text.Trim() + "\\" + listView1.SelectedItems[0].Text;
WordToHtmlFile(docPath);
MessageBox.Show("转换完毕!", "提示信息", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
}
else
{
MessageBox.Show("请选择要转换的Word文档!");
}
}
}
private void button4_Click(object sender, EventArgs e)//批量转换
{
if (listView1.Items.Count > 0)
{
if (MessageBox.Show("确定将批量将文档转换为HTML文件吗?", "提示信息", MessageBoxButtons.OKCancel, MessageBoxIcon.Information) == DialogResult.OK)
{
BatchConvert(txtWordPath.Text.Trim());
}
}
}
private void button3_Click(object sender, EventArgs e)
{
Application.Exit();
}
}
partial class Form1
{
/// <summary>
/// 必需的设计器变量。
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// 清理所有正在使用的资源。
/// </summary>
/// <param name="disposing">如果应释放托管资源,为 true;否则为 false。</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows 窗体设计器生成的代码
/// <summary>
/// 设计器支持所需的方法 - 不要
/// 使用代码编辑器修改此方法的内容。
/// </summary>
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.txtWordPath = new System.Windows.Forms.TextBox();
this.button1 = new System.Windows.Forms.Button();
this.groupBox1 = new System.Windows.Forms.GroupBox();
this.groupBox2 = new System.Windows.Forms.GroupBox();
this.listView1 = new System.Windows.Forms.ListView();
this.button2 = new System.Windows.Forms.Button();
this.button3 = new System.Windows.Forms.Button();
this.statusStrip1 = new System.Windows.Forms.StatusStrip();
this.tsslInfo = new System.Windows.Forms.ToolStripStatusLabel();
this.folderBrowserDialog1 = new System.Windows.Forms.FolderBrowserDialog();
this.button4 = new System.Windows.Forms.Button();
this.groupBox1.SuspendLayout();
this.groupBox2.SuspendLayout();
this.statusStrip1.SuspendLayout();
this.SuspendLayout();
//
// label1
//
this.label1.AutoSize = true;
this.label1.Location = new System.Drawing.Point(8, 21);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(65, 12);
this.label1.TabIndex = 0;
this.label1.Text = "选择目录:";
//
// txtWordPath
//
this.txtWordPath.Location = new System.Drawing.Point(70, 17);
this.txtWordPath.Name = "txtWordPath";
this.txtWordPath.Size = new System.Drawing.Size(294, 21);
this.txtWordPath.TabIndex = 1;
//
// button1
//
this.button1.Location = new System.Drawing.Point(370, 15);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(59, 23);
this.button1.TabIndex = 2;
this.button1.Text = "浏览...";
this.button1.UseVisualStyleBackColor = true;
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// groupBox1
//
this.groupBox1.Controls.Add(this.txtWordPath);
this.groupBox1.Controls.Add(this.button1);
this.groupBox1.Controls.Add(this.label1);
this.groupBox1.FlatStyle = System.Windows.Forms.FlatStyle.System;
this.groupBox1.Location = new System.Drawing.Point(13, 12);
this.groupBox1.Name = "groupBox1";
this.groupBox1.Size = new System.Drawing.Size(435, 44);
this.groupBox1.TabIndex = 3;
this.groupBox1.TabStop = false;
this.groupBox1.Text = "选择目录";
//
// groupBox2
//
this.groupBox2.Controls.Add(this.listView1);
this.groupBox2.Location = new System.Drawing.Point(13, 62);
this.groupBox2.Name = "groupBox2";
this.groupBox2.Size = new System.Drawing.Size(435, 187);
this.groupBox2.TabIndex = 4;
this.groupBox2.TabStop = false;
this.groupBox2.Text = "Word文档";
//
// listView1
//
this.listView1.FullRowSelect = true;
this.listView1.Location = new System.Drawing.Point(6, 17);
this.listView1.Name = "listView1";
this.listView1.Size = new System.Drawing.Size(423, 161);
this.listView1.TabIndex = 0;
this.listView1.UseCompatibleStateImageBehavior = false;
this.listView1.View = System.Windows.Forms.View.List;
//
// button2
//
this.button2.Location = new System.Drawing.Point(225, 259);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(59, 23);
this.button2.TabIndex = 6;
this.button2.Text = "转换";
this.button2.UseVisualStyleBackColor = true;
this.button2.Click += new System.EventHandler(this.button2_Click);
//
// button3
//
this.button3.Location = new System.Drawing.Point(389, 259);
this.button3.Name = "button3";
this.button3.Size = new System.Drawing.Size(59, 23);
this.button3.TabIndex = 7;
this.button3.Text = "取消";
this.button3.UseVisualStyleBackColor = true;
this.button3.Click += new System.EventHandler(this.button3_Click);
//
// statusStrip1
//
this.statusStrip1.Items.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.tsslInfo});
this.statusStrip1.Location = new System.Drawing.Point(0, 285);
this.statusStrip1.Name = "statusStrip1";
this.statusStrip1.Size = new System.Drawing.Size(460, 22);
this.statusStrip1.TabIndex = 8;
this.statusStrip1.Text = "statusStrip1";
//
// tsslInfo
//
this.tsslInfo.Name = "tsslInfo";
this.tsslInfo.Size = new System.Drawing.Size(131, 17);
this.tsslInfo.Text = "toolStripStatusLabel1";
//
// button4
//
this.button4.Location = new System.Drawing.Point(298, 259);
this.button4.Name = "button4";
this.button4.Size = new System.Drawing.Size(75, 23);
this.button4.TabIndex = 9;
this.button4.Text = "批量转换";
this.button4.UseVisualStyleBackColor = true;
this.button4.Click += new System.EventHandler(this.button4_Click);
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 12F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(460, 307);
this.Controls.Add(this.button4);
this.Controls.Add(this.statusStrip1);
this.Controls.Add(this.button3);
this.Controls.Add(this.button2);
this.Controls.Add(this.groupBox2);
this.Controls.Add(this.groupBox1);
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedSingle;
this.MaximizeBox = false;
this.Name = "Form1";
this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
this.Text = "Word转换为Html";
this.groupBox1.ResumeLayout(false);
this.groupBox1.PerformLayout();
this.groupBox2.ResumeLayout(false);
this.statusStrip1.ResumeLayout(false);
this.statusStrip1.PerformLayout();
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label label1;
private System.Windows.Forms.TextBox txtWordPath;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.GroupBox groupBox1;
private System.Windows.Forms.GroupBox groupBox2;
private System.Windows.Forms.Button button2;
private System.Windows.Forms.Button button3;
private System.Windows.Forms.StatusStrip statusStrip1;
private System.Windows.Forms.FolderBrowserDialog folderBrowserDialog1;
private System.Windows.Forms.ListView listView1;
private System.Windows.Forms.Button button4;
private System.Windows.Forms.ToolStripStatusLabel tsslInfo;
}